An entity relationship (ER) diagram (also known as an ERD for Entity-Relationship Diagram) is a visual representation of the relationships between entities within a system. ER Diagrams are often used when designing relational databases. An ERD serves as a blueprint for designing a database by depicting entities, their attributes, and the relationships between them.
ER diagrams help in conceptualizing the structure of a database and serve as a communication tool between database designers, developers, and stakeholders.
Example
Here’s an example of a simple ER diagram:
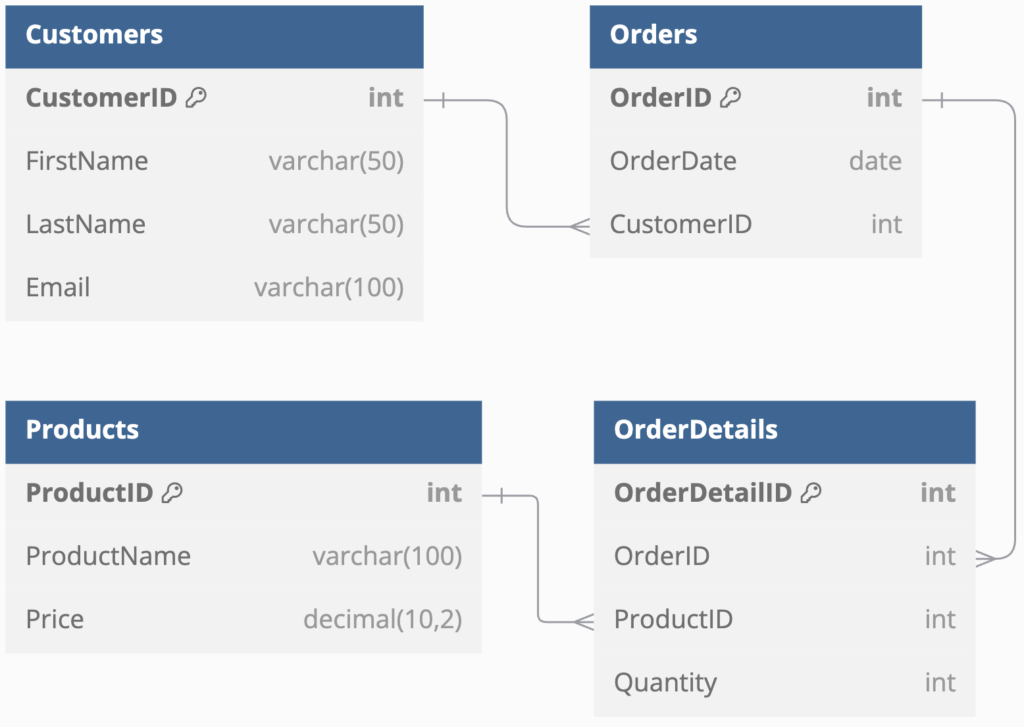
This ER diagram represents a small database schema. We can see each entity and the relationships between them.
In this case, each entity is a separate table, and we’ve also got the column definitions, along with their data types.
Does the Layout Matter?
From a technical perspective, the actual layout of the ER diagram is not important. What I mean is, changing the position of each entity doesn’t change its meaning, so long as the entity relationship is correct, as well as any other attributes/properties listed within the diagram. In other words, it doesn’t matter where each entity is positioned within the ER diagram, as long as it correctly represents the relationships between them.
For example, the above schema could just as easily be represented as follows:

However, from a communication and readibility perspective, the layout can be very important.
The layout of an ER diagram affects the clarity, readability, and overall effectiveness of the diagram as a communication tool. While the core information—entities, attributes, and relationships—remains the same regardless of layout, how you organize these elements can greatly influence how easily others can understand the structure of your database.
Key Considerations for ER Diagram Layout
Here are some things to think about when creating your next ERD:
- Logical Grouping:
- Group related entities close to each other. For example, place the
Orders
,OrderDetails
, andProducts
entities near each other if they are closely related.
- Group related entities close to each other. For example, place the
- Avoid Overlapping Lines:
- Ensure that relationships (lines) between entities do not overlap unnecessarily. Overlapping lines can make the diagram confusing and harder to follow.
- Consistent Direction:
- Try to arrange the diagram so that the flow of relationships follows a consistent direction, such as left-to-right or top-to-bottom. This makes it easier to trace relationships across the diagram.
- Use of Space:
- Use white space effectively to prevent the diagram from appearing cluttered. This helps in distinguishing between different entities and their relationships.
- Hierarchy Representation:
- If there is a clear hierarchy (e.g., a
Customer
entity that leads toOrders
, which leads toOrderDetails
), represent it visually by placing higher-level entities above or to the left of lower-level entities.
- If there is a clear hierarchy (e.g., a
- Labeling:
- Ensure all entities, attributes, and relationships are clearly labeled. Relationship lines should have clear labels (like “1-to-Many” or standard symbols to depict this) to describe the nature of the relationship.
- Avoid Overcrowding:
- If the diagram becomes too complex, consider breaking it into smaller, related sub-diagrams. This can make it easier to focus on specific parts of the database structure.
- Alignment:
- Align entities and attributes neatly to enhance readability. Misaligned elements can make the diagram look unprofessional and harder to interpret.
Benefits of a Good Layout
Putting in the effort to make sure our ER diagrams aren’t a scrambled mess can have many benefits. Some of these include:
- Enhanced Communication: A well-organized layout helps stakeholders quickly grasp the relationships and structure of the database.
- Ease of Maintenance: For ongoing projects, a clear layout makes it easier for developers and database administrators to understand and modify the database.
- Accurate Representation: Proper layout minimizes the risk of misinterpretation, ensuring that the database design is implemented as intended.
Common Layout Styles
Here are some common layouts that you might like to experiment with to see what works best in each situation:
- Top-Down Layout: Where higher-level entities are placed at the top and lower-level entities cascade down.
- Left-to-Right Layout: Where the primary entity starts on the left, and related entities flow to the right.
- Central Hub Layout: Where a central entity (like
Customers
) is placed in the center, with related entities radiating outward.
Here’s an example of a slightly more complex ER diagram that uses many of the above layout principles:
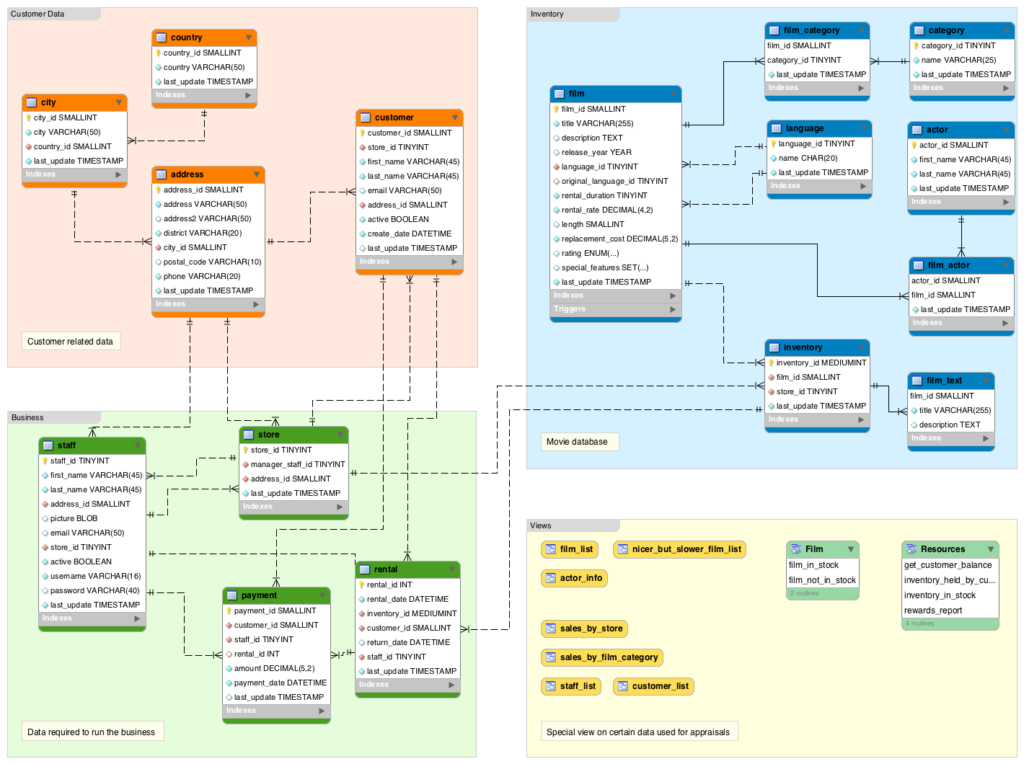
This is the schema for the Sakila sample database, which can be used for learning and testing things out in MySQL.
So to recap, while the layout does not alter the core information of an ER diagram, it significantly impacts how effectively that information is conveyed. A thoughtful, organized layout enhances clarity, reduces misunderstandings, and improves the overall usability of the diagram.
ER Diagrams are Code-Agnostic
It’s worth noting that ER diagram is generally considered code-agnostic. This means that an ER diagram is a conceptual representation of the database structure that is independent of any specific database management system (DBMS) or programming language.
The primary purpose of an ER diagram is to model the entities, attributes, and relationships in a way that is easily understood by humans, regardless of the underlying implementation.
Why ER Diagrams Are Code-Agnostic
Let’s dig a little deeper on this one, and look at some of the main reasons ER diagrams are code-agnostic:
- Conceptual Nature:
- ER diagrams focus on the abstract design of the database, including entities, their attributes, and the relationships between them. They do not include details about the specific syntax or features of any particular DBMS.
- Universality:
- The concepts of entities, relationships, and attributes are universal across relational databases. Whether you are using SQL Server, MySQL, PostgreSQL, Oracle, or another system, these concepts remain the same.
- Flexibility:
- Separation of Design and Implementation:
- The ER diagram serves as a blueprint or design document, while the actual implementation (SQL code, DBML code, etc.) is derived from this design. The design can remain consistent even if the implementation language or platform changes.
Code-Dependent Elements (Not in ER Diagrams)
Certain implementation details that may be DBMS-specific are not always represented in an ER diagram. These could include:
- Data Types:
- In some cases, an ER diagram won’t specify the exact data types used in the database (e.g.,
VARCHAR
,INT
), as these can vary between DBMSs. That said, there are also many cases where data types are specified. Generally, if a project is close to implementation, data types may be specified in order to guide the developers. On the other hand, if the project is still in its early stages, where the main audience is non-technical, data types may be omitted.
- In some cases, an ER diagram won’t specify the exact data types used in the database (e.g.,
- Indexing:
- Indexes, which are critical for performance in many DBMSs, are usually not depicted in ER diagrams.
- Stored Procedures, Triggers, and Functions:
- These are typically not included in ER diagrams, as they are implementation-specific and depend on the particular DBMS.
- Constraints:
- While basic constraints like primary keys and foreign keys may be shown, more complex constraints, such as check constraints or unique constraints, are often left out or only abstractly represented.
How ER Diagrams Translate to Code
When converting an ER diagram into a physical database schema, the following steps typically occur:
- Entity to Table Mapping:
- Each entity in the ER diagram usually corresponds to a table in the database.
- Attribute to Column Mapping:
- Each attribute in the ER diagram becomes a column in the corresponding table.
- Relationships to Keys:
- Relationships are translated into foreign keys and join operations in the database.
- Choice of Data Types and Constraints:
- During implementation, data types are assigned to columns, and various constraints (e.g.,
NOT NULL
,UNIQUE
) are added based on the requirements.
- During implementation, data types are assigned to columns, and various constraints (e.g.,
Example SQL Code
Here’s an example of SQL code that will create the four tables in the above ER diagram, along with the relationships between them:
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Email VARCHAR(100)
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
OrderDate DATE,
CustomerID INT,
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(100),
Price DECIMAL(10, 2)
);
CREATE TABLE OrderDetails (
OrderDetailID INT PRIMARY KEY,
OrderID INT,
ProductID INT,
Quantity INT,
FOREIGN KEY (OrderID) REFERENCES Orders(OrderID),
FOREIGN KEY (ProductID) REFERENCES Products(ProductID)
);
This example should work reasonably well across multiple RDBMSs (with the possible exception of some of the data type syntax). Depending on the RDBMS, we could add more specific instructions that might only be supported in that RDBMS, or perhaps has a different syntax to other RDBMSs. As mentioned, this generally isn’t a concern at the ER diagram level, given it should generally be code-agnostic.
In this example, we use FOREIGN KEY
constraints to establish relationships between the tables. This is quite standard in SQL, but again, each RDBMS has its own nuances when it comes to the specific options available.
Example DBML Code
DBML, which stands for Database Markup Language, is an open-source DSL language designed to define and document database schemas and structures. DBML was created by Holistics, an analytics platform company. It was initially developed for dbdiagram.io, which is an online tool that creates ER diagrams based on DBML code.
We can therefore use DBML to create our ER diagram:
Table Customers {
CustomerID int [pk]
FirstName varchar(50)
LastName varchar(50)
Email varchar(100)
}
Table Orders {
OrderID int [pk]
OrderDate date
CustomerID int [ref: > Customers.CustomerID]
}
Table Products {
ProductID int [pk]
ProductName varchar(100)
Price decimal(10, 2)
}
Table OrderDetails {
OrderDetailID int [pk]
OrderID int [ref: > Orders.OrderID]
ProductID int [ref: > Products.ProductID]
Quantity int
}
In fact, that’s the code I used to create the diagram in the first place.
When to Use ER Diagrams?
ER diagrams are essential during the early stages of database design for the following reasons:
- Conceptual Design: To outline the high-level structure of the database and ensure that all entities and relationships are properly identified.
- Communication: To facilitate discussions between stakeholders, developers, and database designers, making sure everyone has a clear understanding of the database’s structure.
- Documentation: To serve as documentation for the database structure, useful for future maintenance and development.
- Normalization: To help in normalizing the database by visually identifying redundancy and improving the overall design.
Scenarios that Warrant ER Diagrams
Here are some scenarios where we can really benefit from an ER diagram:
- New Project Design: When starting a new software project that requires a relational database, an ER diagram helps to design the database structure from scratch.
- Database Redesign: When refactoring or redesigning an existing database, an ER diagram can help visualize the changes needed and their impact.
- System Integration: When integrating multiple databases or systems, ER diagrams can help map out the relationships and dependencies between different data sources.
- Data Migration: During data migration, ER diagrams can ensure that the data is correctly mapped between the old and new database structures.
- Teaching and Learning: ER diagrams are a great tool for teaching and learning database concepts, making complex relationships easier to understand.
Ways to Generate an ER Diagram
ER diagrams can be created in a number of ways. Here are some of the main methods for generating an ER diagram:
- From Scratch: Developers can use diagramming tools (like Lucidchart, Draw.io, or Microsoft Visio) to manually create an ER diagram during the initial design phase.
- From an Existing Database: Many database management tools (like MySQL Workbench, Microsoft SQL Server Management Studio, or Oracle SQL Developer) can automatically generate ER diagrams by reverse-engineering an existing database schema.
- Using Code: We can use a markup language like DBML to create ER diagrams. Some SQL data modeling tools and ERD generators can create ER diagrams from code-based database definitions.
- Online ERD Tools: Web-based tools like dbdiagram.io or Vertabelo allow developers to design ER diagrams online, often with the capability to import/export database schemas.
Each of these methods can serve a different purpose depending on the stage of development and the specific needs of the project.
Summary
In conclusion, entity relationship (ER) diagrams are a fundamental tool in database design, providing a clear and structured way to visualize the relationships between entities within a system.
By mapping out entities, their attributes, and the connections between them, ER diagrams help in creating a coherent blueprint for database construction and management.