In relational databases, a one-to-one relationship is a relationship between two tables where each record in the first table corresponds to one and only one record in the second table, and vice versa.
This kind of relationship is less common compared to other relationship types, but it can be useful in certain scenarios. The one-to-many and many-to-many relationships are much more common than the one-to-one relationship in SQL.
Example
Let’s consider an example where we have two tables: Person
and Passport
. Each person can have only one passport, and each passport is assigned to only one person.
Schema Diagram
Here’s an entity relationship diagram (ERD) that shows the one-to-one relationship between these two tables:
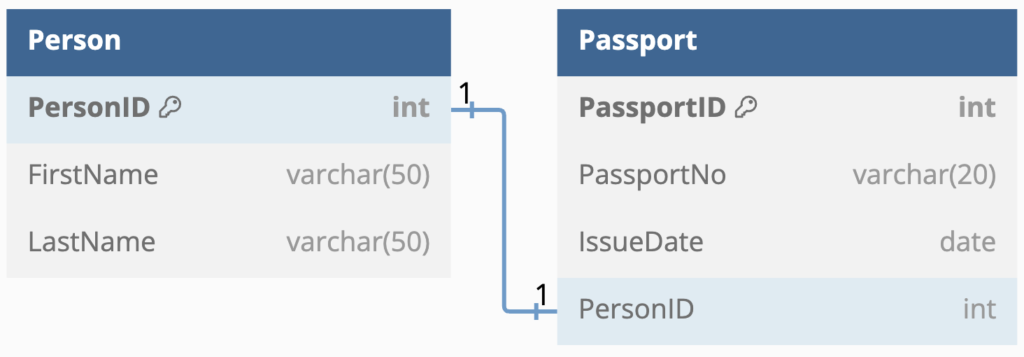
Here, the Person
table has a PersonID
column that’s referenced by the PersonID
column in the Passport
table. The diagram shows a one-to-one relationship by having a 1
against each column reference.
SQL Code
Here’s how we could create these tables in SQL:
CREATE TABLE Person (
PersonID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50)
);
CREATE TABLE Passport (
PassportID INT PRIMARY KEY,
PassportNo VARCHAR(20) UNIQUE,
IssueDate DATE,
PersonID INT UNIQUE,
FOREIGN KEY (PersonID) REFERENCES Person(PersonID) ON DELETE CASCADE
);
Explanation
PersonID
: This is the primary key in thePerson
table.PassportID
: This is the primary key in thePassport
table.PersonID
inPassport
: ThePersonID
in thePassport
table has two constraints:UNIQUE
: Ensures that eachPersonID
in thePassport
table is unique, preventing more than one passport from being associated with the same person.FOREIGN KEY
: This enforces a relationship betweenPerson
andPassport
. In this case we useON DELETE CASCADE
to ensure that if a person is deleted, the corresponding passport record is also deleted, maintaining referential integrity.
What’s the Purpose of a One-to-One Relationship?
One-to-one relationships are relatively rare in database design, but they can be used for the following purposes:
- Storing Optional Data: You want to store data that is relevant only to certain records. Instead of adding nullable columns to a single table, you separate optional data into another table to maintain a clean design.
- Performance Optimization: Large tables can be split into multiple tables to improve performance by reducing the size of frequently accessed data.
- Security: Sensitive data can be stored in a separate table to restrict access.
When to Use a One-to-One Relationship?
Given the nature of one-to-one relationships, you might be struggling to think of a use-case for them. Here are some ideas for scenarios that might warrant a one-to-one relationship:
- Extending Data: If you have a table for
User
and you want to store additional details likeUserProfile
that not every user might have, a one-to-one relationship is suitable. - Specialized Data: You have a
Product
table, and for certain products, you need additional regulatory details. You could have aProductRegulation
table linked one-to-one withProduct
. - Partitioning Data for Security: Sensitive information, such as social security numbers or financial data, might be stored in a separate table to provide stricter access control.
In summary, one-to-one relationships are useful in specific scenarios where data separation, optional data, or security is a concern. They are less common than other relationships but can be useful in the right context.