In SQL, a many-to-many relationship occurs when multiple records in one table can be associated with multiple records in another table.
To represent this type of relationship, a third table—often referred to as a “junction table” or “associative entity”—is used. This junction table typically contains foreign keys that reference the primary keys of the two related tables, allowing for the connection between multiple records.
Example of a Many-to-Many Relationship
Suppose we have two entities: Books
and Authors
. A book can have multiple authors, and an author can write many books. This is a classic example of a many-to-many relationship.
Schema
Here’s an entity relationship (ER) diagram that conveys the many-to-many relationship between the above tables:
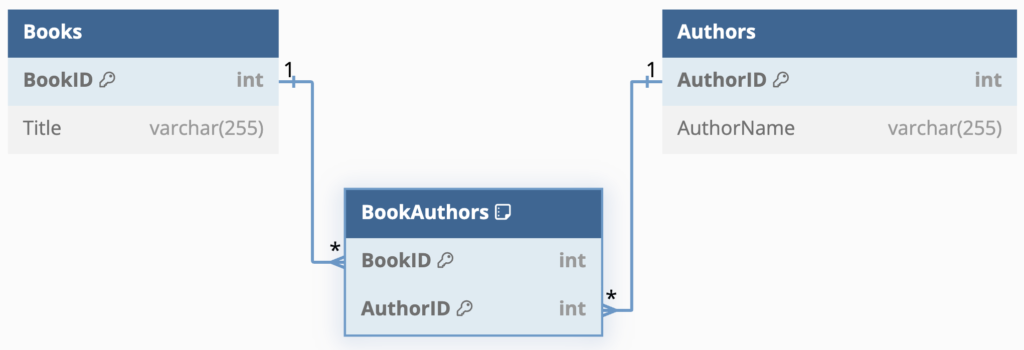
Here, we have a Books
table for all books and an Authors
table for all authors.
But it’s the AuthorsBooks
table that enables the many-to-many relationship. This table has a compound primary key, which contains the combined primary key values from each of the other tables. This effectively links those other tables together. The two columns that make up the compound primary key are also foreign keys, as they’re referencing the values in the other tables’ primary keys.
Specifically, we have a foreign key where the AuthorBooks.AuthorId
column references the Author.AuthorId
column, and we have another foreign key where the AuthorBooks.BookId
column references the Books.BookId
column.
The AuthorsBooks
table is known as a junction table. Junction tables are essential in establishing many-to-many relationships in SQL, due to the fact that the other tables have no way of doing it on their own.
To explain, a foreign key in one table can relate to a primary key in another table, establishing a one-to-one or one-to-many relationship. But when dealing with a many-to-many relationship, a single foreign key isn’t sufficient because one row in a table can be associated with multiple rows in another table, and vice versa. That’s why we use a junction table; we can use a combination of foreign keys to link the primary keys of the related tables.
SQL Code
Here’s an example of code that creates the above schema:
-- Create the Books table
CREATE TABLE Books (
BookID int PRIMARY KEY,
Title nvarchar(255)
);
-- Create the Authors table
CREATE TABLE Authors (
AuthorID int PRIMARY KEY,
AuthorName nvarchar(255)
);
-- Create the AuthorsBooks table
CREATE TABLE BookAuthors (
BookID int,
AuthorID int,
PRIMARY KEY (BookID, AuthorID),
FOREIGN KEY (BookID) REFERENCES Books(BookID),
FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID)
);
Once we’ve run that code, all tables will be created and we’ll have a many-to-many relationship between the Books
and the Authors
tables, as depicted in the above ER diagram.
We can see that the AuthorsBooks
table contains foreign keys that reference the other tables. We can also see that its primary key consists of the respective primary keys for the other tables.
Benefits of Many-to-Many Relationships
Many-to-many relationships offer several benefits when modeling complex data associations. Here’s a breakdown of some of the main benefits:
- Accurate Representation of Real-World Relationships:
- Many real-world scenarios involve entities that naturally relate to each other in a many-to-many fashion. For example, students enrolling in courses or products being included in multiple orders. Using a many-to-many relationship allows our database to mirror these complexities without forcing an unnatural data model.
- Elimination of Redundant Data:
- Without a many-to-many relationship, we might be tempted to include repeated data in one table, leading to redundancy. For example, listing all books an author has written directly in the
Authors
table would involve repeating the authors’ names for each book they’ve written, which increases the chance of data anomalies (e.g., inconsistency, redundancy). Books with multiple authors would further compound this problem. A junction table avoids this issue by normalizing the data.
- Without a many-to-many relationship, we might be tempted to include repeated data in one table, leading to redundancy. For example, listing all books an author has written directly in the
- Referential Integrity:
- Using a many-to-many relationship with foreign keys ensures referential integrity by enforcing valid references between the related tables. For example, the junction table
AuthorsBooks
ensures that only existing authors can be attributed to existing books, maintaining the validity of our data.
- Using a many-to-many relationship with foreign keys ensures referential integrity by enforcing valid references between the related tables. For example, the junction table
- Flexibility in Querying:
- A many-to-many relationship offers flexibility when querying data. We can easily retrieve all the associated records between two tables through the junction table. For instance, we can quickly find all books an author has written or all authors for a particular book with simple
JOIN
operations.
- A many-to-many relationship offers flexibility when querying data. We can easily retrieve all the associated records between two tables through the junction table. For instance, we can quickly find all books an author has written or all authors for a particular book with simple
- Scalability:
- As our dataset grows, a many-to-many relationship scales well, allowing us to manage a large number of associations efficiently. For example, adding a new enrollment for a course could simply involve inserting a row into the junction table (e.g.
Enrollments
) without altering the structure of theStudents
orCourses
tables.
- As our dataset grows, a many-to-many relationship scales well, allowing us to manage a large number of associations efficiently. For example, adding a new enrollment for a course could simply involve inserting a row into the junction table (e.g.
- Reduced Maintenance:
- When using a many-to-many relationship, the data structure is more maintainable. Changes in one entity don’t require restructuring the related data. For example, adding a new course or student doesn’t require updating multiple rows or tables; we only need to insert new entries into the respective tables.
- Support for Complex Queries:
- Many-to-many relationships allow for more complex queries that involve relationships between entities. For instance, we can query which authors wrote books in a specific genre, even if the genre information is stored in a separate table.
When to Use a Many-to-Many Relationship
A many-to-many relationship is used when an association between two entities cannot be represented accurately with just a one-to-one or one-to-many relationship. Here are some more scenarios where we would use a many-to-many relationship:
- Academic Systems: Where students can enroll in multiple courses, and courses can have multiple students.
- E-commerce: Products and orders where each order can contain multiple products, and each product can be part of multiple orders.
- Social Media: Where users can follow multiple other users, and those users can also be followed by multiple other users.
- Library Systems: Where books can be borrowed by multiple members, and members can borrow multiple books.
- Actors and Movies: An actor can appear in multiple movies, and a movie can feature multiple actors.
- Tags and Articles: An article can have multiple tags, and a tag can be associated with multiple articles.
- Employees and Projects: An employee can work on multiple projects, and a project can involve multiple employees.
- Musicians and Bands: A musician can be part of multiple bands, and a band can have multiple musicians.
- Vehicles and Drivers: A vehicle can be driven by multiple drivers, and a driver can operate multiple vehicles.
Using a many-to-many relationship in these scenarios ensures that the database can accurately model the complex associations between records without unnecessary redundancy or data duplication.
By leveraging many-to-many relationships, we ensure that our database design is both efficient and reflective of the real-world scenarios it aims to model, while also promoting data integrity and ease of maintenance.